In programming, loops are used to repeat a block of code. For example, if we want to show a message 100 times, then we can use a loop. It's just a simple example, we can achieve much more with loops.
In the previous tutorial, we learned about Python for loop. Now we will learn about the while
loop.
Learn Python with Challenges
Solve challenges and become a Python expert.
Python while Loop
Python while
loop is used to run a block code until a certain condition is met.
The syntax of while
loop is:
while condition:
# body of while loop
Here,
- A
while
loop evaluates thecondition
- If the
condition
evaluates toTrue
, the code inside thewhile
loop is executed. condition
is evaluated again.- This process continues until the condition is
False
. - When
condition
evaluates toFalse
, the loop stops.
Flowchart of Python while Loop
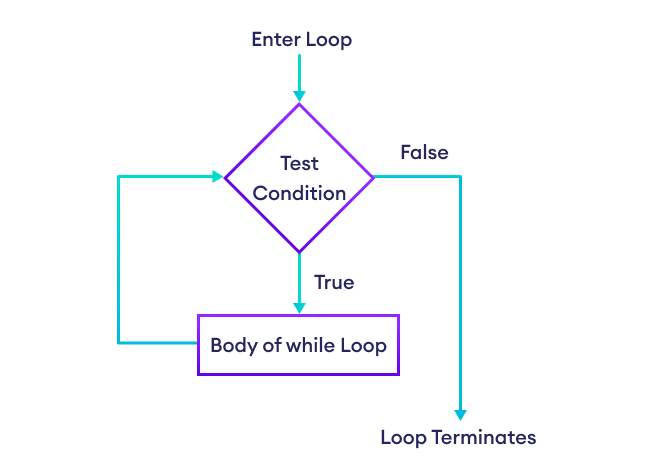
Example: Python while Loop
# program to display numbers from 1 to 5
# initialize the variable
i = 1
n = 5
# while loop from i = 1 to 5
while i <= n:
print(i)
i = i + 1
Output
1 2 3 4 5
Here's how the program works:
Variable | Condition: i <= n |
Action |
---|---|---|
i = 1 n = 5 |
True |
1 is printed. i is increased to 2. |
i = 2 n = 5 |
True |
2 is printed. i is increased to 3. |
i = 3 n = 5 |
True |
3 is printed. i is increased to 4. |
i = 4 n = 5 |
True |
4 is printed. i is increased to 5. |
i = 5 n = 5 |
True |
5 is printed. i is increased to 6. |
i = 6 n = 5 |
False |
The loop is terminated. |
Example 2: Python while Loop
# program to calculate the sum of numbers
# until the user enters zero
total = 0
number = int(input('Enter a number: '))
# add numbers until number is zero
while number != 0:
total += number # total = total + number
# take integer input again
number = int(input('Enter a number: '))
print('total =', total)
Output
Enter a number: 12 Enter a number: 4 Enter a number: -5 Enter a number: 0 total = 11
In the above example, the while
iterates until the user enters zero. When the user enters zero, the test condition evaluates to False
and the loop ends.
Infinite while Loop in Python
If the condition
of a loop is always True
, the loop runs for infinite times (until the memory is full). For example,
age = 32
# the test condition is always True
while age > 18:
print('You can vote')
In the above example, the condition
always evaluates to True
. Hence, the loop body will run for infinite times.
Python While loop with else
In Python, a while
loop may have an optional else
block.
Here, the else
part is executed after the condition of the loop evaluates to False
.
counter = 0
while counter < 3:
print('Inside loop')
counter = counter + 1
else:
print('Inside else')
Output
Inside loop Inside loop Inside loop Inside else
Note: The else block will not execute if the while loop is terminated by a break statement.
counter = 0
while counter < 3:
# loop ends because of break
# the else part is not executed
if counter == 1:
break
print('Inside loop')
counter = counter + 1
else:
print('Inside else')
Output
Inside loop Inside else
Python for Vs while loops
The for
loop is usually used when the number of iterations is known. For example,
# this loop is iterated 4 times (0 to 3)
for i in range(4):
print(i)
The while
loop is usually used when the number of iterations is unknown. For example,
while condition:
# run code until the condition evaluates to False