Python break Statement
The break
statement is used to terminate the loop immediately when it is encountered.
The syntax of the break statement is:
break
Learn Python with Challenges
Solve challenges and become a Python expert.
Working of Python break Statement
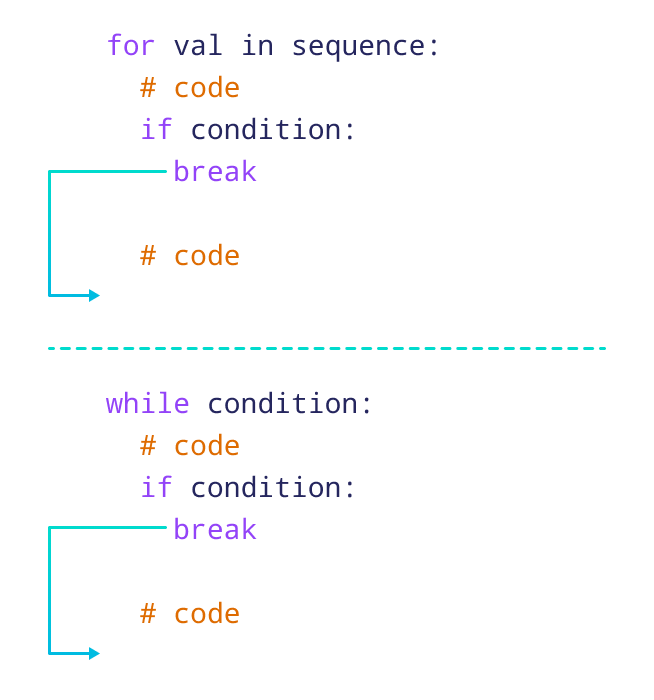
The working of break statement in for loop and while loop is shown above.
Python break Statement with for Loop
We can use the break
statement with the for
loop to terminate the loop when a certain condition is met. For example,
for i in range(5):
if i == 3:
break
print(i)
Output
0 1 2
In the above example, we have used the for
loop to print the value of i
. Notice the use of the break
statement,
if i == 3:
break
Here, when i
is equal to 3, the break
statement terminates the loop. Hence, the output doesn't include values after 2.
Note: The break
statement is almost always used with decision-making statements.
Python break Statement with while Loop
We can also terminate the while
loop using the break statement. For example,
# program to find first 5 multiples of 6
i = 1
while i <= 10:
print('6 * ',(i), '=',6 * i)
if i >= 5:
break
i = i + 1
Output
6 * 1 = 6 6 * 2 = 12 6 * 3 = 18 6 * 4 = 24 6 * 5 = 30
In the above example, we have used the while
loop to find the first 5 multiples of 6. Here notice the line,
if i >= 5:
break
This means when i
is greater than or equal to 5, the while
loop is terminated.
Python continue Statement
The continue
statement is used to skip the current iteration of the loop and the control flow of the program goes to the next iteration.
The syntax of the continue
statement is:
continue
Working of Python continue Statement
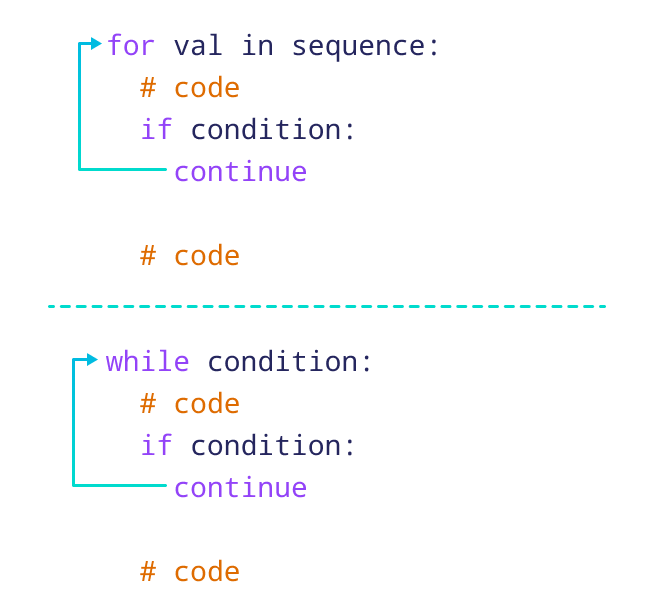
The working of the continue
statement in for and while loop is shown above.
Python continue Statement with for Loop
We can use the continue
statement with the for
loop to skip the current iteration of the loop. Then the control of the program jumps to the next iteration. For example,
for i in range(5):
if i == 3:
continue
print(i)
Output
0 1 2 4
In the above example, we have used the for
loop to print the value of i
. Notice the use of the continue
statement,
if i == 3:
continue
Here, when i
is equal to 3, the continue
statement is executed. Hence, the value 3 is not printed to the output.
Python continue Statement with while Loop
In Python, we can also skip the current iteration of the while loop using the continue statement. For example,
# program to print odd numbers from 1 to 10
num = 0
while num < 10:
num += 1
if (num % 2) == 0:
continue
print(num)
Output
1 3 5 7 9
In the above example, we have used the while
loop to print the odd numbers between 1 to 10. Notice the line,
if (num % 2) == 0:
continue
Here, when the number is even, the continue statement skips the current iteration and starts the next iteration.